Simulation Definition
Once you have defined a geometry, assigned materials, and specified sources and receivers, you are now ready to create a SimulationDefinition
.
A Treble Simulation
is created from an instance of this class, or a list of instances of the SimulationDefinition
, which have been added to the project.
A SimulationDefinition
contains the following required properties:
Property | Type | Description | Example value |
---|---|---|---|
name | string | A human-friendly name for identifying the simulation later. | "LectureHall_01" |
simulation_type | SimulationType | Defines which solver will be used in the simulation. | treble.SimulationType.dg , treble.SimulationType.ga , treble.SimulationType.hybrid |
model | ModelObj | The model that has been processed and added to the project. | |
crossover_frequency | int | The frequency, in Hertz, defining the domain of the simulation types. | 1000 |
ir_length | float | The duration, in seconds, of the output simulation impulse response. | 0.7 |
energy_decay_threshold | float | The magnitude of energy decay, in dB, after which the simulation will stop calculating. | 35 |
receiver_list | list(ModelObj) | A list containing the desired Treble receivers. | |
source_list | list(ModelObj) | A list containing the desired Treble sources. | |
material_assignment | list(MaterialAssignment) | A list containing the material assignments for each unique layer in the model. |
The model, source and receiver lists, and material assignment lists should all have been assigned prior to creating a simulation definition. To review the process of creating these lists, please refer to the documentation.
The properties of ir_length
and energy_decay_threshold
are both Termination Criteria, which you can read more about in our technical reference. It is not necessary to define both: simply choose the termination criteria that is most appropriate for your application, and define this property.
In addition to the required fields, the following properties may be optionally assigned during intialisation.
Property | Type | Description | Example value |
---|---|---|---|
simulation_settings | SimulationSetting | Optional. Custom simulation settings. See Solver settings. | |
on_task_error | SimulationOnTaskError | Optional. Define on task error behaviour. Defaults to "ignore". | treble.SimulationOnTaskError.ignore , treble.SimulationOnTaskError.stop_project , `treble.SimulationOnTaskError.stop_simulation |
description | str | Optional description of simulation. | "LoudspeakerA_angle+_15_degrees" |
Create a simulation definition
The following code examples creates two simulations with nearly identical simulation definitions. The only difference between the two examples is the choice of termination criteria used by the calculator.
Example: Create a simulation definition using the energy decay termination criterion
The following code shows an example simulation definition using the energy decay threshold for the termination criteria. The simulation will continue to compute until the global energy decay curve (EDC) has lost 35 dB from its starting value across all octave bands. This allows for the calculation of from the resulting impulse according to the standard framework. Using the energy decay threshold is a good option when the reverberation time of the model is not yet known (as in the case of bulk simulations), or when the assumptions supporting a Sabine absorption estimate are not fulfilled (, unevenly distributed absorption coeffiecients or a model with a small internal volume).
sim_def = treble.SimulationDefinition(
name="My simulation", # unique name of the simulation
simulation_type=treble.SimulationType.hybrid, # the type of simulation
model=room, # a room created in an earlier step
crossover_frequency=720, # which frequency the GA solver takes over from the DG solver
energy_decay_threshold=35, # cut off the simulation when the energy decay curve reaches -35 dB
receiver_list=[rec_1, rec_2], # two previously defined receivers
source_list=[source], # one previously defined source
material_assignment=material_assignment # the previously defined material assignment list
)
Example: Create a simulation definition using the duration of the impulse response
The following code defines the same simulation as before, using the duration of the impulse response rather than the energy decay curve as the termination criterion.
The simulation will continue until it has calculated the time steps making up the value of ir_length
,
where is the duration ir_length
in milliseconds, and is the simulation sampling rate in Hertz.
This approach allows for precise control over the length of resulting time-domain results, and is a good option when you already know the length of the reverberation time in the model, or are only interested in simulating the early reflections of a model.
You must take care when using this termination criteria, as room acoustic parameters will not return valid results if the simulation duration (ir_length
) has been set shorter than the duration required for the impulse response to decay to zero.
For instance, if ir_length=0.1
in a model where seconds, most acoustic parameters calculations on the simulation output will be invalid.
sim_def = treble.SimulationDefinition(
name="My simulation",
simulation_type=treble.SimulationType.hybrid,
model=room,
crossover_frequency=720,
ir_length=0.1, # cut off the simulation at 0.1 seconds long
receiver_list=[rec_1, rec_2],
source_list=[source],
material_assignment=material_assignment
)
The simulation definition has not yet been added to the project at this point! You can still check and modify details of the simulation definition prior to uploading it, at which point the metadata associated with the simulation is no longer mutable.
Example: modify the termination criteria after creating the simulation definition
As with other objects within the SDK, the properties of the simulation defintion may be accessed after creating it.
The following example creates a simulation definition, then modifies the energy_decay_threshold
property.
# create a simulation definition
sim_def = treble.SimulationDefinition(
name="My simulation", # unique name of the simulation
simulation_type=treble.SimulationType.hybrid, # the type of simulation
model=room, # a room created in an earlier step
crossover_frequency=720, # which frequency the GA solver takes over from the DG solver
energy_decay_threshold=35, # cut off the simulation when the energy decay curve reaches -35 dB
receiver_list=receivers, # two previously defined receivers
source_list=source, # two previously defined sources
material_assignment=material_assignment # the previously defined material assignment list
)
# change the energy decay threshold
print(sim_def.energy_decay_threshold)
sim_def.energy_decay_threshold = 70
print(sim_def.energy_decay_threshold)
Use the definition to create a simulation
After the simulation definition has been created, you may now create the simulation object and add it to the project. This is done by calling the following method:
simulation = project.add_simulation(sim_def)
Now, all the information required to run the simulation has been uploaded to the servers and stored for future use.
Methods
A simulation definition is an intermediary step to creating a simulation in the project. Prior to uploading the definition, or after retrieving the definition of a previous simulation, there are a number of methods to interact with the object.
.plot()
Plot the simulation definition, including the geometry, sources, and receivers.
Arguments
None.
Returns
An interactive, three-dimensional plot of the simulation geometry.
Example
sim_def.plot()
.remove_invalid_receivers()
Remove receivers falling outside the domain of the geometry
Arguments
None.
Returns
None.
Example
sim_def.remove_invalid_receivers()
# verify if the receiver list has been updated.
print(len(sim_def.receiver_list))
print(len(receivers))
.remove_invalid_sources()
Remove sources falling outside the domain of the geometry.
Arguments
None.
Returns
None.
Example
sim_def.remove_invalid_sources()
# verify if the source list has been updated.
print(len(sim_def.source_list))
print(len(source))
.validate()
Validates the simulation definition, checking for valid source and receiver positions inside the model and far enough away from the boundaries, and verifies that all layers in the model have materials assigned to them.
Arguments
None.
Returns
Validation object.
Example
validation = sim_def.validate()
# display the results of the validation phase
dd.display(validation)
Examples
Validate and fix a simulation definition
The following example shows a simple simulation validation workflow. In this case, the model has been defined as a narrow shoebox room, with one valid and one invalid source and receiver.
sim_def = treble.SimulationDefinition(
name="My simulation", # unique name of the simulation
simulation_type=treble.SimulationType.hybrid, # the type of simulation
model=room, # a room created in an earlier step
crossover_frequency=720, # which frequency the GA solver takes over from the DG solver
energy_decay_threshold=35, # cut off the simulation when the energy decay curve reaches -35 dB
receiver_list=receivers, # two previously defined receivers
source_list=source, # two previously defined sources
material_assignment=material_assignment # the previously defined material assignment list
)
sim_def.plot()
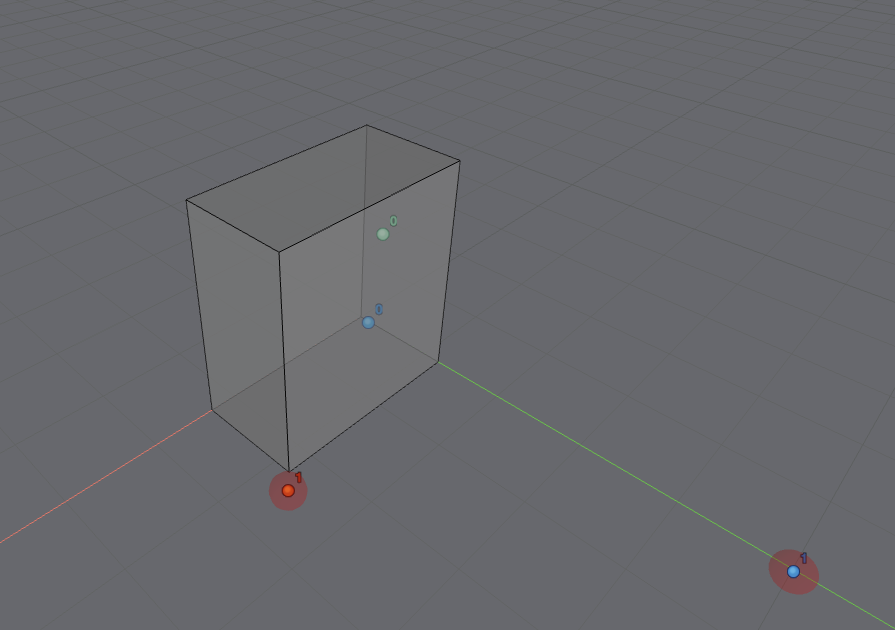
A source and a receiver in this plot are highlighted in red, indicating that they are the invalid sources. The details of the validation can be printed, which identifies the type of problem related with receiver 2 and source 2.
validation = sim_def.validate()
dd.display(validation)
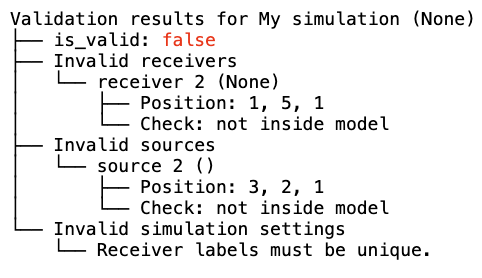
Instead of manually changing the source and receiver lists and either changing them in place or creating a new simulation definition, we can instead call the helper functions to trim the source and receiver lists.
sim_def.remove_invalid_sources()
sim_def.remove_invalid_receivers()
sim_def.plot()
Plotting again, we can confirm that the updates have been correctly applied.
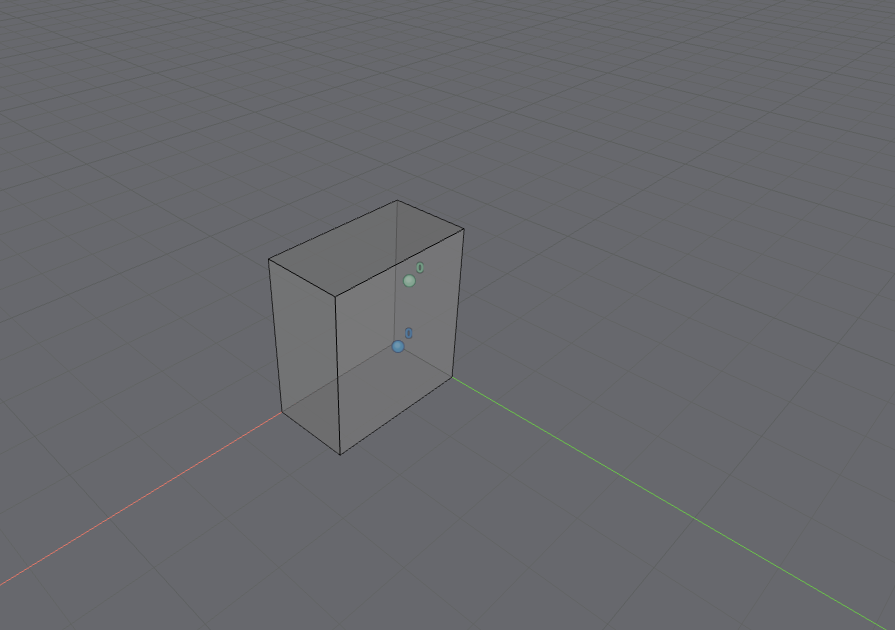