Create Room
The Treble SDK comes with a selection of geometry generation functions which may be used to programmatically generate simple rooms with code. These functions may be used to define custom shoebox, angled shoebox, L-shaped, T-shaped, U-shaped, and polygon rooms with a simple input in two dimensions.
In all cases, the footprint of the room is defined in two dimensions, along with an offset amount by which the shape is extruded into three dimensions.
To work with these functions, you must first create a model or set of models using one of the methods described below, and then add the model or models to the project.
Methods
create_shoebox_room
The simplest geometry handled by this module is a 6-sided shoebox room. This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
width_x | float | The width, in meters, of the room. | 2.3 |
depth_y | float | The depth, in meters, of the room. | 1.2 |
height_z | float | The height, in meters, of the room. | 2.5 |
join_wall_layers | boolean | Description | True , False |
The join_wall_layers boolean determines if each wall within the shoebox will be assigned the same material, or if each wall should be assigned a materially individually.
The simplest model possible with this method is when join_wall_layers is True
.
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates a shoebox room with joined wall layers.
# Create a narrow and tall shoebox room
room = treble.GeometryDefinitionGenerator.create_shoebox_room(
width_x=2.3,
depth_y=1.2,
height_z=2.5,
join_wall_layers=True,
)
# visually confirm that there are three separate layers to the model
room.plot()
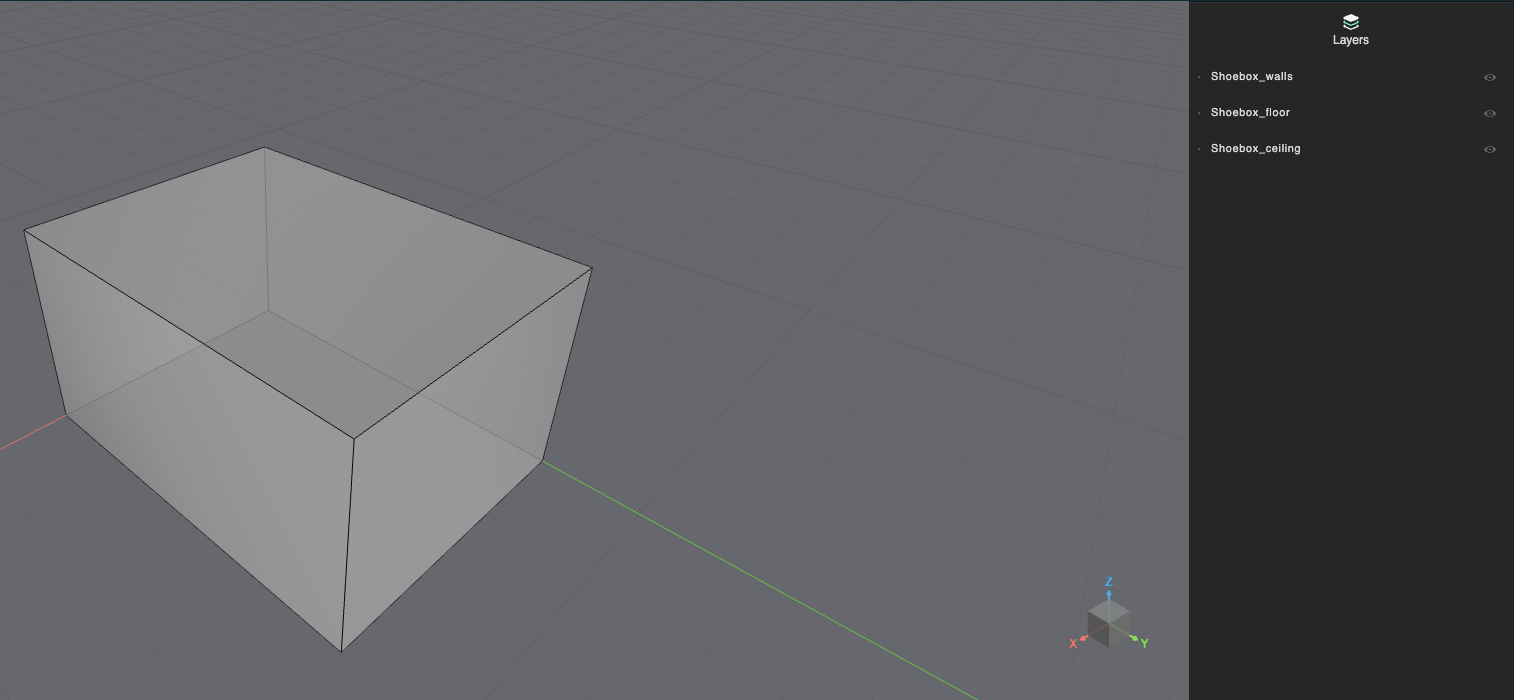
When you plot the room model, the individual layers and their associated layer names are displayed as a list on the right-hand-side of the display. This lis confirms that only three unique layers are associated with this model object: The floor, the ceiling, and the walls.
In contrast, the following code snippet generates an identical shoebox room with separated layers, with a total of six unique layers.
# Create a narrow and tall shoebox room
room = treble.GeometryDefinitionGenerator.create_shoebox_room(
width_x=2.3,
depth_y=1.2,
height_z=2.5,
join_wall_layers=False,
)
# visually confirm that there are six separate layers to the model
room.plot()
create_random_shoebox_rooms
Create multiple random shoebox rooms. The dimensions are randomly drawn from the specified range using uniform probability.
Property | Type | Description | Example value |
---|---|---|---|
n_rooms | int | Number of random rooms to create. | 20 |
x_range | tuple[float,float] | The width, in meters, of all generated rooms are randomly drawn from this range. | [5,15] |
y_range | tuple[float,float] | The depth, in meters, of all generated rooms are randomly drawn from this range. | [6,12] |
z_range | tuple[float,float] | The height, in meters, of all generated rooms are randomly drawn from this range. | [2,4] |
join_wall_layers | boolean | Description | True , False |
Returns
list[GeometryDefinition
] objects, which must be then added to the models contained within the project.
Example
The following code example creates 20 random shoebox rooms.
# Create a list of random shoebox rooms
rooms = treble.GeometryDefinitionGenerator.create_random_shoebox_rooms(
n_rooms=20,
x_range=[5,15],
y_range=[6,12],
z_range=[2,4],
join_wall_layers=False)
rooms[0].plot()
create_angled_shoebox_room
The simplest geometry handled by this module is a 6-sided shoebox room with angled walls, which may be either a parrallelogram or a trapezoid, depending on the provided parameters.
This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
base_side | float | The width, in meters, of the side of the room at y=0 | 5 |
depth_y | float | The depth, in meters, of the room. | 1.2 |
left_angle | float | The internal angle, in degrees, between the vertical walls at p(x,y) = (0,0) | 45 |
right_angle | float | The external angle, in degrees, between the vertical walls at p(x,y) = (base_side,0) | 100 |
height_z | float | The height, in meters, of the room. | 100 |
join_wall_layers | boolean | Description | True , False |
The properties of this function are illustrated below:
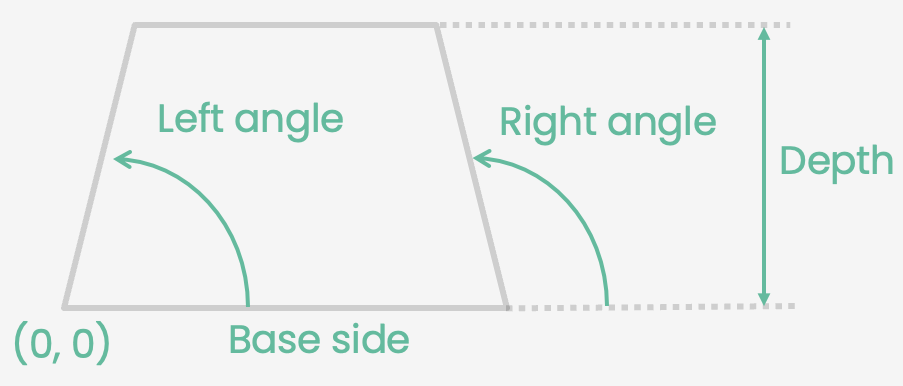
The function always assumes two walls, both parallel to the x-axis.
It will automatically generate side walls at the specified angles to connect the parallel walls, and will scale the wall at y=depth_y
to be the appropriate length.
This function will not generate a valid room if right_angle
and left_angle
are defined such that the sum of all angles exceeds 360°. Likewise, if depth_y
is defined such that the two side walls will converge a y < depth_y
, the function will not generate a valid room.
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates a trapezoidal shoebox room with joined wall layers.
# Create an angled shoebox room (trapezoid)
room = treble.GeometryDefinitionGenerator.create_angled_shoebox_room(
base_side=5,
depth_y=3,
left_angle=45,
right_angle=100,
height_z=2.2,
join_wall_layers=True
)
room.plot()
create_random_angled_shoebox_rooms
Create multiple random angled-shoebox rooms. The dimensions are randomly drawn from the specified ranges.
This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
n_rooms | int | Number of random rooms to create. | 20 |
base_side_range | tuple[float,float] | The width, in meters, of all generated rooms are randomly drawn from this range. | [3,8] |
deth_range | tuple[float,float] | The depth, in meters, of all generated rooms are randomly drawn from this range. | [2,7] |
left_angle_range | tuple[float,float] | The left angles (in degrees) for all generated rooms are randomly drawn from this range. | [15,90] |
right_angle_range | tuple[float,float] | The right angles (in degrees) for all generated rooms are randomly drawn from this range. | [15,160] |
height_range | tuple[float,float] | The height, in meters, of all generated rooms are randomly drawn from this range. | [2,4] |
join_wall_layers | boolean | Description | True , False |
Returns
list[GeometryDefinition
] objects, which must be then added to the models contained within the project.
Example
The following code example creates 20 random angled-shoebox rooms.
# Create random angled shoebox rooms
rooms = treble.GeometryDefinitionGenerator.create_random_angled_shoebox_rooms(
n_rooms=20,
base_side_range=[3,8],
depth_range=[2,7],
left_angle_range=[15,90],
right_angle_range=[15,160],
height_range=[2,4],
join_wall_layers=False)
rooms[0].plot()
create_L_shaped_room
The L-shaped room creates a room with 8 total surfaces, where the arms of the L-shape are determined by four unique values. The four values specify the lengths of the line segments defining the concave edges of the L-shape, as illustrated in the following image.
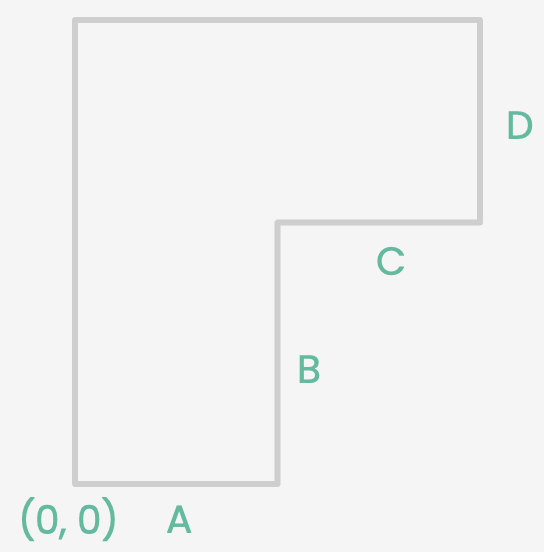
Property | Type | Description | Example value |
---|---|---|---|
a_side | float | The length of side A in meters. | 1 |
b_side | float | The length of side B in meters. | 1.1 |
c_side | float | The length of side C in meters. | 2 |
d_side | float | The length of side D in meters. | 1 |
height_z | float | The height of the room in meters. | 2.2 |
join_wall_layers | boolean | Description | True , False |
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates an L-shaped room with joined wall layers.
# Create an L-shaped room
room = treble.GeometryDefinitionGenerator.create_L_shaped_room(
a_side=1,
b_side=2,
c_side=3,
d_side=2,
height_z=2.2,
join_wall_layers=True
)
room.plot()
create_random_L_shaped_rooms
Create multiple random L-shaped rooms. The dimensions are randomly drawn from the specified ranges.
This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
n_rooms | int | Number of random rooms to create. | 20 |
a_side_range | tuple[float,float] | The length of side A of all generated rooms are randomly drawn from this range. | [1,5] |
b_side_range | tuple[float,float] | The length of side B of all generated rooms are randomly drawn from this range. | [2,8] |
c_side_range | tuple[float,float] | The length of side C of all generated rooms are randomly drawn from this range. | [7,9] |
d_side_range | tuple[float,float] | The length of side D of all generated rooms are randomly drawn from this range. | [1,10] |
height_range | tuple[float,float] | The height, in meters, of all generated rooms are randomly drawn from this range. | [2,4] |
join_wall_layers | boolean | Description | True , False |
Returns
list[GeometryDefinition
] objects, which must be then added to the models contained within the project.
Example
The following code example creates 20 random L-shaped rooms.
# Create random L-shaped rooms
rooms = treble.GeometryDefinitionGenerator.create_random_L_shaped_rooms(
n_rooms=20,
a_side_range=[1,5],
b_side_range=[2,8],
c_side_range=[7,9],
d_side_range=[1,10],
height_range=[2,4],
join_wall_layers=False)
rooms[0].plot()
create_T_shaped_room
The T-shaped room creates a room with 10 total surfaces, where the arms of the T-shape are determined by four unique values. The four values specify the lengths of certain line segments along the edges of the T-shape, as illustrated in the following image.
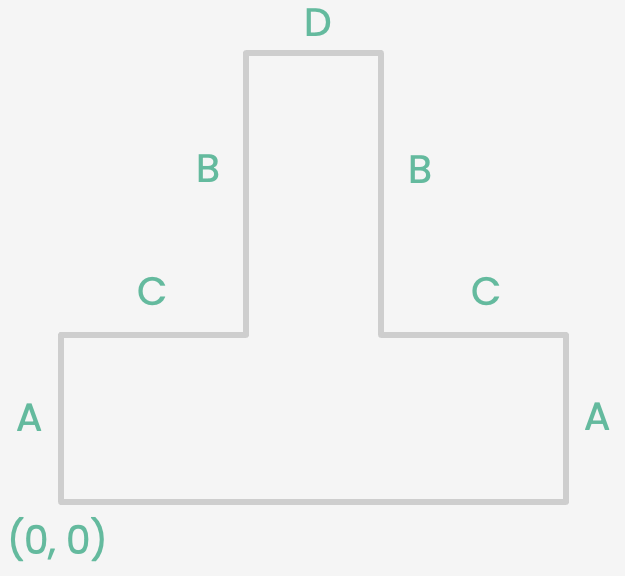
Property | Type | Description | Example value |
---|---|---|---|
a_side | float | The length of side A in meters. | 1 |
b_side | float | The length of side B in meters. | 3 |
c_side | float | The length of side C in meters. | 1 |
d_side | float | The length of side D in meters. | 2 |
height_z | float | The height of the room in meters. | 2.2 |
join_wall_layers | boolean | Description | True , False |
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates an T-shaped room with separated wall layers.
# Create an T-shaped room
room = treble.GeometryDefinitionGenerator.create_T_shaped_room(
a_side= 1,
b_side= 3,
c_side= 1,
d_side= 2,
height_z= 2.2,
join_wall_layers= False
)
room.plot()
create_random_T_shaped_rooms
Create multiple random T-shaped rooms. The dimensions are randomly drawn from the specified ranges.
This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
n_rooms | int | Number of random rooms to create. | 20 |
a_side_range | tuple[float,float] | The length of side A of all generated rooms are randomly drawn from this range. | [1,5] |
b_side_range | tuple[float,float] | The length of side B of all generated rooms are randomly drawn from this range. | [2,8] |
c_side_range | tuple[float,float] | The length of side C of all generated rooms are randomly drawn from this range. | [7,9] |
d_side_range | tuple[float,float] | The length of side D of all generated rooms are randomly drawn from this range. | [1,10] |
height_range | tuple[float,float] | The height, in meters, of all generated rooms are randomly drawn from this range. | [2,4] |
join_wall_layers | boolean | Description | True , False |
Returns
list[GeometryDefinition
] objects, which must be then added to the models contained within the project.
Example
The following code example creates 20 random T-shaped rooms.
rooms = treble.GeometryDefinitionGenerator.create_random_T_shaped_rooms(
n_rooms=20,
a_side_range=[1,5],
b_side_range=[2,8],
c_side_range=[7,9],
d_side_range=[1,10],
height_range=[2,4],
join_wall_layers=False)
rooms[5].plot()
create_U_shaped_room
The U-shaped room creates a room with 10 total surfaces, where the dimensions of the U-shape are determined by four unique values. The four values specify the lengths of certain line segments along the edges of the U-shape, as illustrated in the following image.
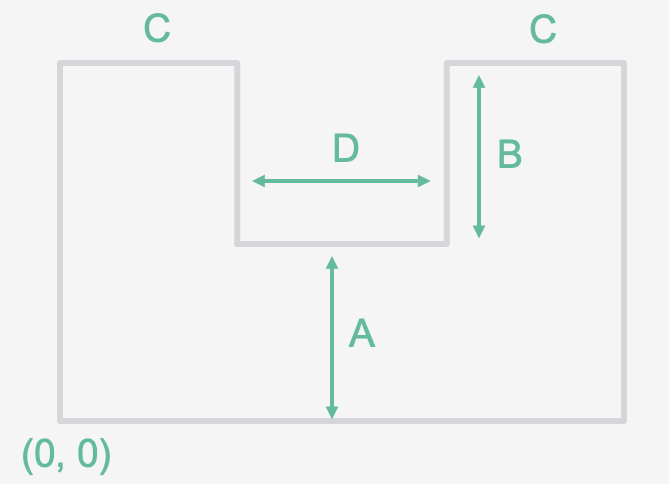
Property | Type | Description | Example value |
---|---|---|---|
a_side | float | The length of side A in meters. | 1 |
b_side | float | The length of side B in meters. | 3 |
c_side | float | The length of side C in meters. | 1 |
d_side | float | The length of side D in meters. | 2 |
height_z | float | The height of the room in meters. | 2.2 |
join_wall_layers | boolean | Description | True , False |
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates an U-shaped room with separated wall layers.
# Create an U-shaped room
room = treble.GeometryDefinitionGenerator.create_U_shaped_room(
a_side= 1,
b_side= 3,
c_side= 1,
d_side= 2,
height_z= 2.2,
join_wall_layers= False
)
room.plot()
create_random_U_shaped_rooms
Create multiple random U-shaped rooms. The dimensions are randomly drawn from the specified ranges.
This function takes the following arguments:
Property | Type | Description | Example value |
---|---|---|---|
n_rooms | int | Number of random rooms to create. | 20 |
a_side_range | tuple[float,float] | The length of side A of all generated rooms are randomly drawn from this range. | [1,5] |
b_side_range | tuple[float,float] | The length of side B of all generated rooms are randomly drawn from this range. | [2,8] |
c_side_range | tuple[float,float] | The length of side C of all generated rooms are randomly drawn from this range. | [7,9] |
d_side_range | tuple[float,float] | The length of side D of all generated rooms are randomly drawn from this range. | [1,10] |
height_range | tuple[float,float] | The height, in meters, of all generated rooms are randomly drawn from this range. | [2,4] |
join_wall_layers | boolean | Description | True , False |
Returns
list[GeometryDefinition
] objects, which must be then added to the models contained within the project.
Example
The following code example creates 20 random U-shaped rooms.
rooms = treble.GeometryDefinitionGenerator.create_random_U_shaped_rooms(
n_rooms=20,
a_side_range=[1,5],
b_side_range=[2,8],
c_side_range=[7,9],
d_side_range=[1,10],
height_range=[2,4],
join_wall_layers=False)
rooms[0].plot()
create_polygon_room
The final method of the Geometry Generator uses a list of points defined in x- and y- dimensions to create the footprint of a room.
Property | Type | Description | Example value |
---|---|---|---|
points_xy | List[float] | The list of points on the x-y plane creating the footprint, defined in meters. | [[6.4, 2.2], [4.2, 4.4], [2.8, 6.6]] |
height_z | float | The height of the room in meters. | 2.2 |
join_wall_layers | boolean | Description | True , False |
The list of points should contain a series of 2D points, represented as a pair of coordinates [x,y]
.
These points define the vertices of the polygon shape.
This function constructs the wall by connecting the points sequentially: while you may reverse the entire order of the list and still yield a valid input, arbitrary reordering of individual points within the list may break the continuity of the closed perimeter, resulting in a non-water-tight model.
Always ensure that the consecutive points in the list form the continuous edge of the desired shape. Verify the points visually if possible, or confirm that they form a closed loop!
Returns
Model
object, which must be then added to the models contained within the project.
Example
The following code example creates two valid rooms and one invalid room.
# define the hexagon points in a counter-clockwise direction
edge_points_hexagon= [[4.2, 4.4],[6.4, 2.2],[2.8, 6.6],[0.2, 4],[0, 3.4],[0.7, 1.9],[2.4, 0.6]]
room1 = treble.GeometryDefinitionGenerator.create_polygon_room(
points_xy=edge_points_hexagon,
height_z=2.2,
join_wall_layers=False
)
room1.plot()
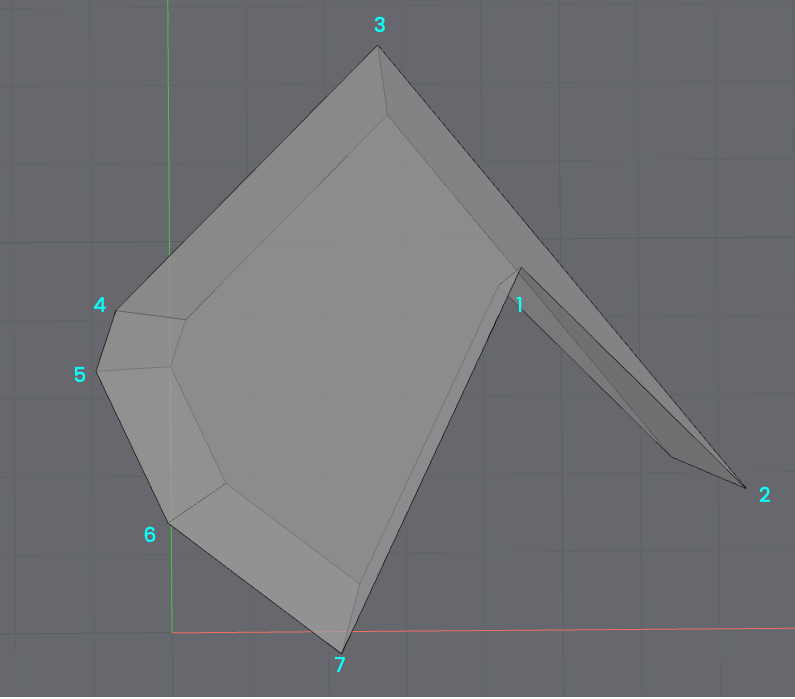
flipped_edge_points_hexagon = edge_points_hexagon[::-1] # flip the order of the original edge_points_hexagon list
room = treble.GeometryDefinitionGenerator.create_polygon_room(
points_xy=flipped_edge_points_hexagon,
height_z=2.2,
join_wall_layers=False
)
room2.plot()
print(flipped_edge_points_hexagon) # confirm that the order is flipped
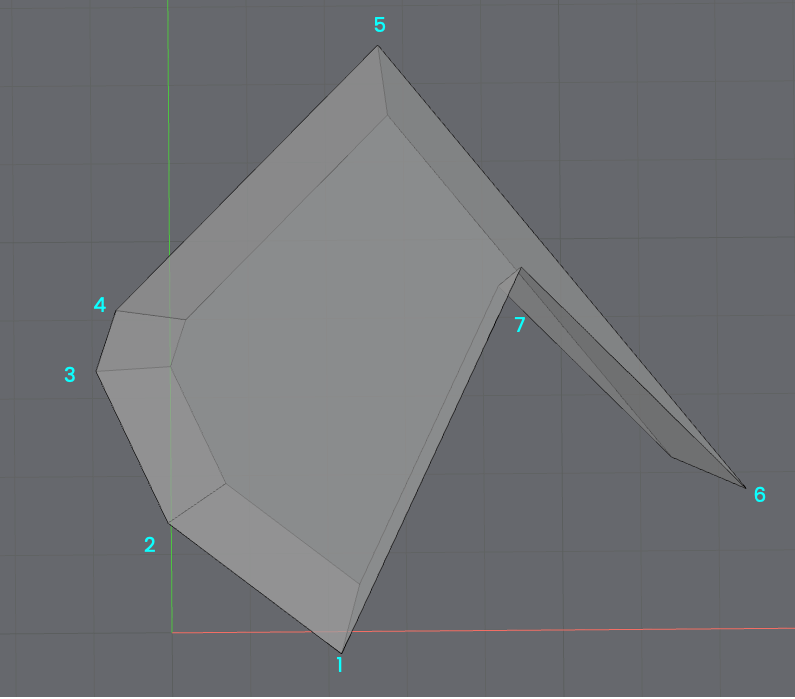
Now, we will switch the order of the elements at index 1 and index 2 of the flipped vector, and plot the resulting room.
# switch the elements
old_index_1 = flipped_edge_points_hexagon[1]
old_index_2 = flipped_edge_points_hexagon[2]
flipped_edge_points_hexagon[1] = old_index_2
flipped_edge_points_hexagon[2] = old_index_1
room3 = treble.GeometryDefinitionGenerator.create_polygon_room(
points_xy=flipped_edge_points_hexagon,
height_z=2.2,
join_wall_layers=False,
)
room3.plot()
print(flipped_edge_points_hexagon)
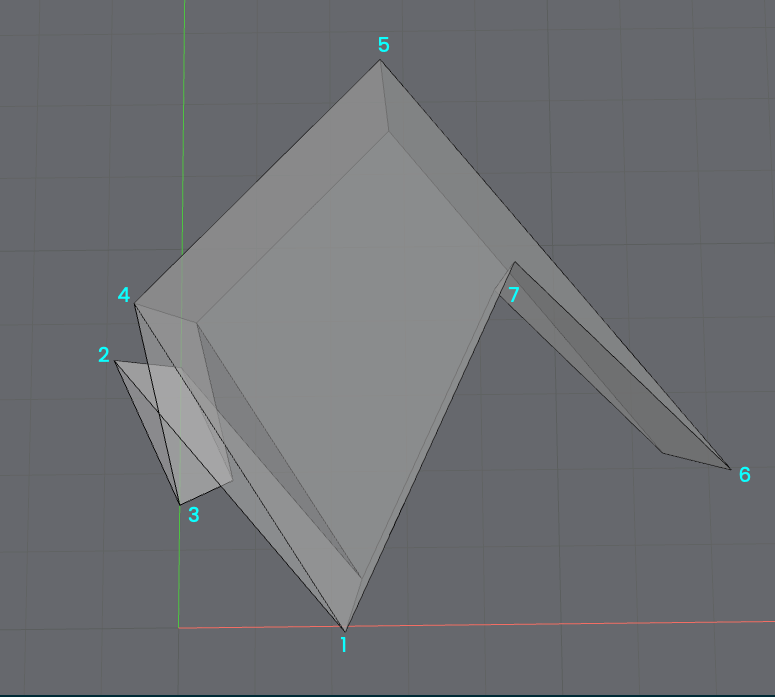
As can be seen in the bad example, the room will still generate with the invalid point order, but this model will return a MesherError
later in the process, during the Setting up Compute step.
Adding a model to the project
Once you have created the model using the GeometryDefinitionGenerator
, you may add the model to the project for use in simulations using the project.add_model
method.
# create the room
room = treble.GeometryDefinitionGenerator.create_shoebox_room(
width_x=2.3,
depth_y=1.2,
height_z=2.5,
join_wall_layers=False,
)
# add the project and define the name
project.add_model(model_name='test shoebox 1',model_file_path=room)
models = project.get_models()
dd.display(models)
You cannot have multiple models with identical names within the same project! If you run project.add_model()
and another model with the same name already exists, you will be prompted to choose a new name!