Generic DRTF Import
When importing a device related transfer function (DRTF) we support the import of raw data from either measurements or simulations.
The process is quite simple and just requires a few definitions.
Here we provide an example of importing raw DRTF data from an hdf5
file but the file format is irrelevant, it might as well be a sofa
, mat
, netCDF
or even csv
or txt
.
As long as the file contains the right data and you can parse it from the file, you can import the DRTF.
The example file we have is structured like this:
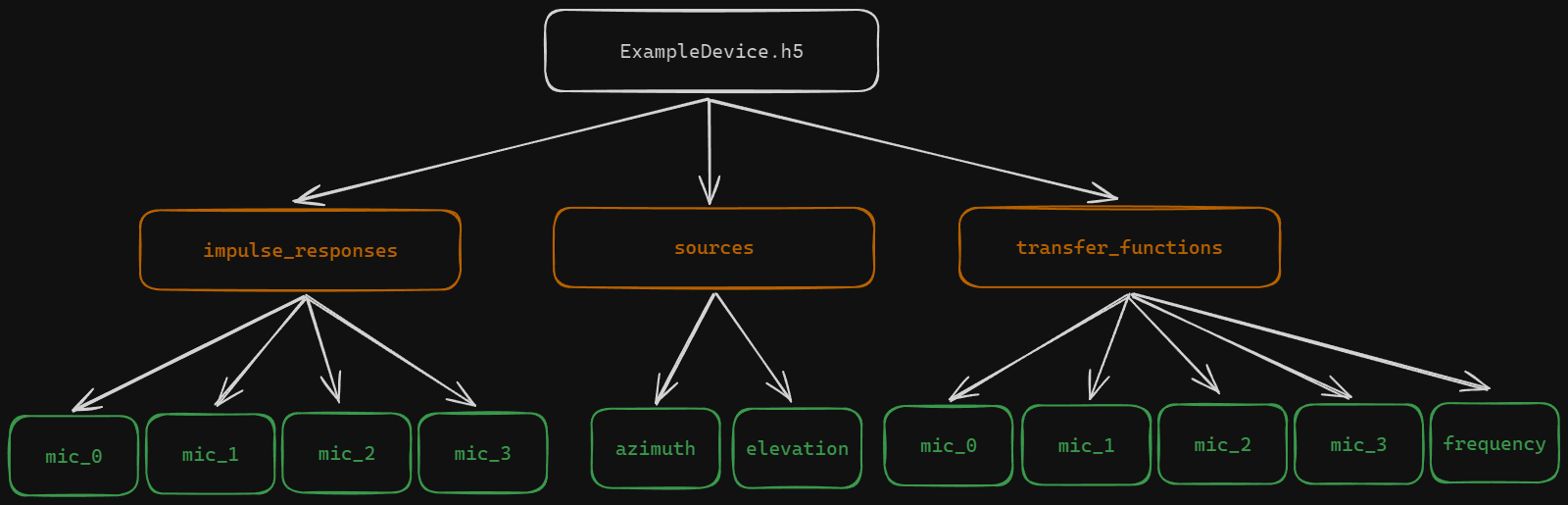
The essential things to define are the source locations on the measurement sphere and either impulse responses or transfer functions of each associated source to a microphone on the device.
Each microphone impulse response or transfer function in the file has an array of size number_of_sources x timesteps/frequencies
.
From a file with such a structure the device can be imported with a simple script showing both option 1 of importing impulse responses and option 2 of importing transfer functions:
import h5py
with h5py.File("ExampleDevice.h5", "r") as f:
sources = f["sources"]
sl = treble.DeviceSourceLocations(
azimuth_deg=sources["azimuth"][:], elevation_deg=sources["elevation"][:]
)
dev_microphones = []
# Here we have two options.
# Option 1, import impulse responses:
irs = f["impulse_responses"]
# Option 2, import transfer functions
tfs = f["transfer_functions"]
for n in range(4):
# Option 1
ir = treble.DeviceImpulseResponses(
impulse_responses=irs[f"mic_{n}"][:], sampling_rate=32_000
)
dev_microphones.append(
treble.DeviceMicrophone(
source_locations=sl, recordings=ir, max_ambisonics_order=16
)
)
# Option 2
tf = treble.DeviceTransferFunctions(
transfer_functions=tfs[f"mic_{n}"][:],
frequency_array=tfs["frequency"][:],
sampling_rate=32_000,
)
# dev_microphones.append(treble.DeviceMicrophone(source_locations=sl, recordings=tf, max_ambisonics_order=16))
device_definition = treble.DeviceDefinition(
device_microphones=dev_microphones,
name="DeviceExample",
description="Device showcase",
)
device = tsdk.device_library.add_device(device_definition=device_definition)
Now the device has been added to the device library and can be used to render a device response from a spatial impulse response. The device can always be fetched by calling:
device = tsdk.device_library.get_by_name("DeviceExample")